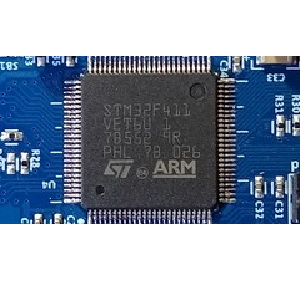
Description
Mastering Embedded ‘Systems programming’ course on ARM Cortex-M4. An in-depth review of the ARM Cortex M4 architecture and systems programming with the STM32F411 Discovery board with the GNU ARM Cross toolchain. Understanding the programmers model, IDE (Integrated Development Environment). Detailed discussions, in-depth study of datasheets and reference manuals, demos and case studies on memory access and its problems (various methods such as macros with offsets and structures), assembly and linking process, I/O subsystem, interrupt (both non-floating and non-floating). floating point) and clock tree configuration with an in-depth discussion of PLL circuitry and performance. Slide shows, code guides, whiteboards, and troubleshooting sessions are an integral part of the course. There are specific issues in embedded systems, especially for advanced microcontrollers, that pose unique challenges.
- The process of manipulating memory directly on a microcontroller either through macros or through structure pointers
- Although pointers for embedded systems look similar to their workstation counterparts, there are subtle differences that can break a program if not handled carefully.
- In embedded firmware development, since program efficiency, determinism, energy efficiency, memory size, writing efficient C programs, and tracking compiled assembly code from C/C++ are of great importance.
- The interrupt handling process is complex, and the generated stack frames differ between implementations such as floating-point stacking and floating-point stacking and unstacking. Interrupt preemption is a complex feature and must be used carefully.
- The Linking and Make process is also highly misunderstood. The subject of object files, portability, and icon resolution pose unique challenges, and consolidated information about them is scarce.
- Object file formats are also a gray area that can cause a lot of confusion when understanding the linking process. Different tables in object file like symbol table, displacement table are difficult to understand without proper guidance.
- I/O pin configuration also presents challenges if the electricity and circuitry of the pins is not well understood. There are several configurations in which each pin of a microcontroller can operate, such as open drain, pull-up, high impedance, etc.
- The clock tree is also very complex for a high-end microcontroller. Multiple clock sources feeding clocks to different subsystems make the initial learning curve very steep. Beyond that, high-frequency clocks are driven by PLLs, which are complex circuits themselves and require a good deal of understanding when configuring them.
- Initializing the processor can be tricky, especially the part where the memory is reset after each reset. Understanding the .text, .data, and .bss sections and setting them up correctly at startup presents unique challenges.
In this course I try to address all the above challenges with theory, diagrams, animation and code. There are many demos to explain difficult concepts. I cleared all the concepts through extensive debugging sessions in the IDE while inspecting the various subsystems of the controller in real-time. I have taken the following steps to resolve the challenges
- Accessing memory and operations in various ways such as macros and structures. I developed concepts gradually until I arrived at the final form of phrases. I have shown examples of incorrect memory access via structures and their reduction. Real-time bitwise operations are checked in the debugger.
- To understand the linking process, I created code samples and first manually compiled each source file and looked at the ELF object files, symbol tables, and displacement tables along with disassembly. When files are compiled into a single object file by the linker, symbol resolution and code and symbol displacement are clearly explained by looking at the final object file. The linker script file is explained in detail with diagrams before and after initialization of the memory.
- An extensive debugging session of the startup process that explains how the processor adjusts memory in real time. The reset controller is described and illustrated in C. The process of initializing the .data segment in RAM and initializing the .bss segment and stack is shown in full detail.
- The Make process is explained using several Makefiles, each one increasing in complexity slightly, until the final make-file is written and fully explained. The build dependency diagram is explained and many possible error scenarios in make are discussed to explain how Makefiles deal with them. This is a comprehensive and competent behavior of Make that helps developers write templates for deeply embedded systems.
- Interrupt handling is shown in detail along with theory. Representation and theory of interrupt handling and preemption for non-floating and floating-point configurations. Concepts such as lazy stacking in the debugger are explained and demonstrated.
- The clock tree is explained in detail. PLL calculations for frequency tuning. Theory of operation of PLL with explanation of various subsystems of PLL like voltage controlled oscillator, frequency divider, phase frequency detector, low pass filter with pictures, animations and timing diagrams. Show the initialization of registers in the clock tree subsystem in the debugger.
- I/O circuit and then I/O pin configuration. All pin modes are described, such as push-pull, open-drain, analog, and alternate functions.
What you will learn in the Mastering Embedded ‘Systems programming’ course on ARM Cortex-M4
-
Get to know the ARM Cortex-M4 Core in the STM32F411 Discovery Board. Data sheets and reference manuals
-
Learn standalone (no IDE required) use of GNU ARM’s embedded toolchain
-
Cube IDE (with Makefile projects) and CUBE Programmer
-
Basics of ARM GNU Debugger (ARM GDB) commands with examples
-
Embedded C for 32-bit controllers. Different methods of registration and memory access, bit operations, inline assembly
-
Learn how to use the CMSIS memory map header
-
Immerse yourself in the clock subsystem, learn about PLL operation, bare metal clock tree and PLL configuration, without using any configuration tools.
-
Understand the interrupt mechanism in detail through extensive demos. Understand stack creation and teardown for both floating and non-floating point modes
-
Disable preemption with examples running on the debugger
-
Lots of additional information on topics like lazy stacking, double word padding, memory barrier instructions, etc. are provided with demos.
-
Learn how to set up an exception handling table from the reference guide. Learn the difference between exceptions and interrupts
-
Learn how to copy initial variables from Flash to SRAM during startup. Set stack and bss memory areas with examples and live memory views when debugging
-
Set and configure SysTick and EXTI interrupts
-
Understand the ELF object file format. Look at the object files via the objdump (for ARM) and readelf commands to check the ELF format in detail.
-
A deep dive into the linker mechanism. Learn about symbol displacement and resolution by checking symbol tables, displacement tables, and more.
-
Understanding how global variables are handled by the compiler and linker. Know the properties of linker variables and how to use them
-
VMA (virtual memory address) and LMA (load memory address). Understand how VMA and LMA are controlled through linker scripts
-
Write linker scripts for various memory configurations that derive from knowledge of the linker mechanism
-
Understand the Make mechanism through multiple examples of increasing complexity. Understand how a dependency tree works
-
Write relatively complex Makefiles
-
Familiarize yourself with the GPIO subsystem, the I/O pins circuit. Learn different pin modes such as open drain, push pull, alternate functions and internal pull
This course is suitable for people who
- Graduates of computer science, electrical and electronic sciences and recent graduates looking for a career in embedded systems
- Working professionals looking to upgrade to an ARM Cortex-M4 controller from their 8-bit or 16-bit counterparts.
- Enthusiasts and those interested in programming Lamb of Steel in STM32F4 microcontroller
Mastering Embedded ‘Systems programming’ course specifications on ARM Cortex-M4
- Publisher: Udemy
- teacher: Diptopal Basu
- Training level: beginner to advanced
- Training duration: 27 hours and 29 minutes
- Number of courses: 58
Headlines of Mastering Embedded ‘Systems programming’ course on ARM Cortex-M4 on 8/2023
Prerequisites of Mastering Embedded ‘Systems programming’ course on ARM Cortex-M4
- Basic microcontroller knowledge is a must, at least knowledge of any 8-bit controller, better still if you are aware of any 16-bit architecture
- Good knowledge of Binary and Hexadecimal number systems is a must
- Intermediate knowledge of C programming and basic pointer, data structure and bitwise operator concepts are a must
- Knowledge of embedded C is not needed, as necessary embedded C will be tackled in the course itself
- Knowledge of basic analog and digital electronics is good to have
- STM32F411 Discovery Board and Mini USB cable needed to follow the course
Course images
Sample video of the course
Installation guide
After Extract, view with your favorite Player.
Subtitle: None
Quality: 720p
download link
File(s) password: www.downloadly.ir
Size
14.9 GB
Be the first to comment