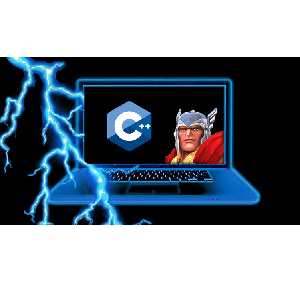
Description
The C++ Programming Language: Learn and Master C++ course. The goal of this course is to make C++ programming an easy-to-learn and accessible subject for programmers at any level. Even if you don’t know how to program or have no experience using C++, it’s not a problem! I designed this course to take you from zero to champion in C++ and explain the concepts from the ground up. We will start by downloading Visual Studio Code IDE (free) and writing a hello world program in C++. At the end of the course you will be comfortable working with advanced data structures such as trees and linked lists and writing algorithms to work with them. You will have basic programming skills and the ability to solve coding interview level questions using C++ code. In this course, we will cover the following:
- C++ Basics – including how to run a C++ program, import header files, internal data types, and output information to the console using C++
- Input, conditional statements, and loops – such as if statements, switch statements, loops, and while loops in C++
- User-defined types – such as structs, classes, and enums in C++
- Intermediate C++ – Includes Standard Library, Date and Time in C++, Recursion, REGEX, Linked Lists, and Trees in C++
- Containers – such as vectors, leading lists, lists, deques, collections, maps, stacks, queues and how to work with each of these using C++ code
- Object Oriented Programming (OOP) – includes classes, objects and inheritance in C++
Algorithms taught in this course: along with an explanation of the algorithm, a hands-on opportunity to practice implementing it, and a step-by-step explanation showing how to code the solution using C++.
- String Algorithms – Frequently asked questions in coding interviews about strings in C++:
- Roman to integer
- palindrome
- Reverse characters
- Valid parenthesis
- Valid anagram
- Array and Vector Algorithms – Frequently asked questions in coding interviews about arrays in C++:
- Delete element
- Remove duplicates from the sorted array
- Pascal’s triangle
- Sort array by equality
- Tree Algorithms – Frequently asked questions in coding interviews about trees in C++:
- Inorder navigation
- Pre-order navigation
- Mail order navigation
- Maximum depth (height) of the binary tree
- Balanced binary tree
- Total path
- Breadth First Search (BFS)
- Depth First Search (DFS)
- Linked List Algorithms – Frequently asked questions in coding interviews about Strings in C++:
- Merge two sorted linked lists
- Remove linked list elements
- Backlink list
- Search and Sorting Algorithms – mastering the main search and sorting algorithms in computer science:
- Introduction section
- Linear search
- Binary search
- Sort selection
- Bubble sort
- Quick sort
- Merge sort
- Insert sort
- root sorting
- stack sort
- Shell sorting
New sections added to the course:
- Abstraction and Resource Management – Learn how to manage resources in your C++ programs
- builders
- destroyers
- range resolution operator (::)
- Abstract classes and virtual functions
- Generics, parameter types, and function patterns
- Creative Design Patterns – Master the Gang of Four Design Patterns used to create objects and how to implement them using C++ code.
- Abstract factory
- Manufacturer
- Factory method
- prototype
- singleton
- Structural Design Patterns – Master the Gang of Four Design Patterns used to compose larger, more complex objects and how to implement them using C++ code.
- adapter
- Bridge
- composite
- decorator
- view
- Flight weight
- proxy
- Behavioral Design Patterns – Mastering the Gang of Four Design Patterns used to define how objects interact and how to implement them using C++ code.
- Chain of responsibility
- Command
- Translator
- pointer
- mediator
- souvenir
- Observer
- state of
- Strategy
- Format
- Method
- Visitor
What you will learn in The C++ Programming Language: Learn and Master C++ course
-
Go from little/no experience in C++ to proficiency in writing programs in C++ code
-
C++ Programming Master with a hands-on approach focused on gaining professional C++ experience that you can use to get a job!
-
Understand all 23 group of 4 design patterns and how to implement each of them in C++ in an easy to understand way.
-
Understand important coding concepts like RECURSION, REGEX, LINKED LISTS and TREES with C++ code.
-
Includes interactive tests and coding exercises to ensure you get practical coding knowledge and retain the concepts taught
-
Gain knowledge of C++ CONTAINERS such as vectors, forward lists, lists, stacks, collections, maps, stacks and queues
-
Build coding skills and a deep understanding of computer science, data structures, and algorithms to ace coding interviews at top tech companies!
-
Teaching how to use C++ to code STRING algorithms, such as Roman coding interview questions to integers, palindromes and anagrams
-
Code array algorithms such as element removal, duplicate removal, Pascal’s triangle, and array sorting based on equality.
-
Mastery of trees and coding algorithms such as BREADTH FIRST SEARCH and DEPTH FIRST SEARCH
-
Brush up on your knowledge of LINKED LISTS and learn how to merge linked lists, remove elements from them, and reverse a linked list.
-
Familiarity with SEARCHING and SORTING algorithms and how to implement them using C++ code
This course is suitable for people who
- Anyone who wants to learn and master the C++ programming language
Course specifications The C++ Programming Language: Learn and Master C++
- Publisher: Udemy
- teacher: Robert Gioia
- Training level: beginner to advanced
- Training duration: 7 hours and 57 minutes
- Number of courses: 209
Course headings
Prerequisites of The C++ Programming Language: Learn and Master C++ course
- A computer with Windows, Mac, or Linux
- Eagerness and willingness to learn C++ programming
- Note: All software and programs for this course are FREE
Course images
Sample video of the course
Installation guide
After Extract, view with your favorite Player.
English subtitle
Quality: 720p
download link
File(s) password: www.downloadly.ir
Size
3.1 GB
Be the first to comment